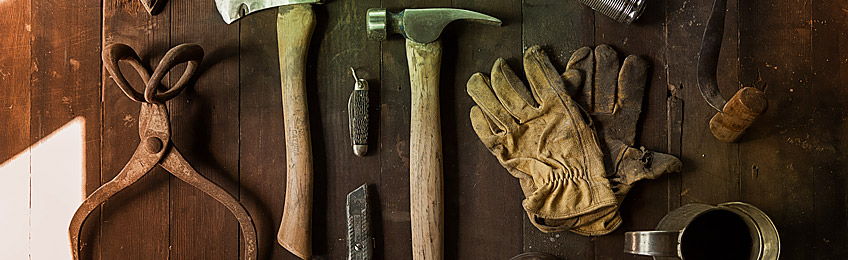
A big part of the front-end in a CMS is to get single pages and/or collections of pages for display or processing. In Kaliko CMS this is handled by the class pageFactory.
If it's one class that you should learn when working with this system it's KalikoCMS.PageFactory
. It provides everything from functions for fetching pages in all kind of directions to events to wire up against to learn when a page is saved.
Short summary of the functions
- DeletePage(Guid pageId)
- Delete the page identified by the id. (In reality only flags it as deleted in the database and is excluded from being read when indexing the site. If you wish to "rescue" an erroneously deleted page you can do so by removing the delete date from it and all pages that is under it and restart the site).
- FindPage(string pageUrl, IRequestManager requestManager)
- Used by the system to find the correct page based on a specific Url. There should be no need to use this function manually.
- GetAncestors(CmsPage page)
- Get a collection with all ancestors to a page. Usefull for bread crumbs and to traverse through parents. Related to GetPagePath but without the current page in the list.
- GetAncestors(Guid pageId)
- Get a collection with all ancestors to a page. Usefull for bread crumbs and to traverse through parents. Related to GetPagePath but without the current page in the list.
- GetChildrenForPage(Guid pageId, Predicate<PageIndexItem> match)
- Gets all children under a particular page that matches the predicate. This can be used to only list pages that should be visible in a menu, or that matches any other criteria.
This function returns aPageCollection
which can be itterated through or further filtered and sorted. The difference by using the predicate as a parameter rather than a where-clause on the collection is that it will perform on a simpler structure and thus be more efficient. - GetChildrenForPage(Guid pageId, PublishState pageState = PublishState.Published)
- An overloaded variant that either returns all children for a particular page or all children for the same page but matching the specified publish state.
- GetChildrenForPageOfPageType(Guid pageId, Type pageType, PublishState pageState = PublishState.Published)
- This function will get all children for a specified page that are of a specified page type. This function is usefull if you got mixed content under the same parent.
- GetChildrenForPageOfPageType(Guid pageId, int pageTypeId, PublishState pageState = PublishState.Published)
- The same as above but using an id to identify the page type rather than the actual type.
- GetPage<T>(Guid pageId)
- Get a typed version of a specified page. This is usefull if you know what type of page you are getting. If you don't you should go with the more generic
GetPage(Guid pageId)
described below. - GetPage(Guid pageId)
- Get a specified page. This will return a
CmsPage
object so you will not find it's properties strongly typed. Instead you can access them using the objectsProperty
collection (myPage.Property["MyPropertyName"]
). - GetPageIdFromUrl(string url)
- Gets the id for a page based on the specified url.
- GetPagePath(CmsPage page)
- Gets a collection of the specified page and all it's ancestors. Usefull for bread crumbs and to traverse through parents.
- GetPagePath(Guid pageId)
- Same as above but with a specified page id instead of the actual page.
- GetPages(Predicate<PageIndexItem> match)
- Get all pages based on a specified predicate.
- GetPages(int pageTypeId, PublishState pageState)
- Get all pages of a specific page type and publish state.
- GetPages(Type pageType, PublishState pageState)
- Get all pages of a specific page type and publish state.
- GetPageTreeFromPage(Guid pageId, Predicate<PageIndexItem> match)
- Get a
PageCollection
of all pages under the specified page matching the given criteria. Great to do filters over the whole site or a particular section. Like getting all blog posts or news articles regardless if they are located on the same level or not. - GetPageTreeFromPage(Guid pageId, PublishState pageState)
- Same as above but criteria is always a publish state.
- GetPageTreeFromPage(Guid rootPageId, Guid leafPageId, PublishState pageState)
- Get a
PageCollection
of all children of the page id specified as root and all pages that is in the same path as the leaf page id. This is ideal for page trees where only the current page path should be opened. - GetParentAtLevel(Guid pageId, int level)
- Get the specified page's parent from the defined level. For instance if you call this function with a page that is 5 levels beyond the root and request the second level page the system will itterate through the parents until it's on level 2 and return that page.
- GetSpecificVersion(Guid pageId, int version)
- Get a specific version of a page.
- GetSpecificVersion(Guid pageId, int languageId, int version)
- Get a specific version of a page.
- GetWorkingCopy(Guid pageId)
- Gets or creates a new working copy version of a page.
- MovePage(Guid pageId, Guid targetId)
- Move the specified page to the new parent specified as target.
- ReorderChildren(Guid pageId, Guid parentId, int position)
- Reorder children when the parent page is set to sort its children using SortIndex.
Events
There are two events that are exposed through KalikoCMS.PageFactory
- one raised when pages are saved (PageEventHandler PageSaved
), one when pages are published (PageEventHandler PagePublished
) and one when pages are deleted (PageEventHandler PageDeleted
).
If you need to be notified when either of the two events occur you can simply create an event handler and attach:
PageFactory.PageSaved += PageFactory_PageSaved; private void PageSavedHandler(object sender, KalikoCMS.Events.PageEventArgs e) { var nameOfPageThatWasSaved = e.Page.PageName; }
The PageEventArgs
that is passed to the handler includes the page id and the actual page that has been saved.